Activity 4: Create Basic Filter
Now that we understand more about pixels and images, we can start to learn how to design your own filter on the image. Let us see some examples on how to design a basic filter on your image.
Example for blue filter
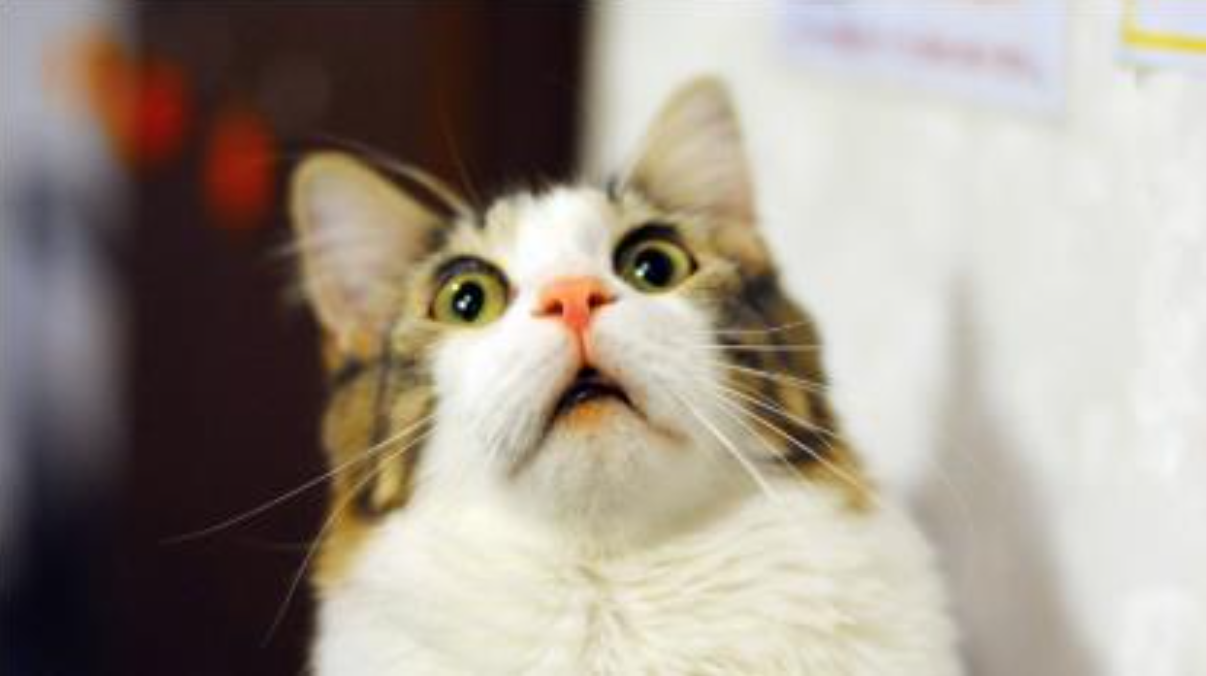
# We need to import PIL package to allow manipulation with pixels.
from PIL import Image
# Open the cat image
img = Image.open("cat.png")
# Let's add the blue filter
for i in range(img.size[0]): # For every column
for j in range(img.size[1]): # For every row
color = img.getpixel( (i,j) )
img.putpixel((i,j),(0, 0, color[2])) # Set the color accordingly
#Save the cat after filtering
img.save("Mycat.png")
Wow! This is our cat after the blue filter.
How did this work? Let’s look at the loop:
for i in range(img.size[0]): # For every column
for j in range(img.size[1]): # For every row
color = img.getpixel( (i,j) ) # Get the current pixel
img.putpixel((i,j),(0, 0, color[2])) # Set the color accordingly
We start by going through the image, by each column and each row, to get each pixel. It then gets the current color value of the pixel. To set a blue filter to that pixel, all we’re doing is setting the ‘Red’ and ‘Green’ RGB values to 0. Therefore, only the ‘Blue’ values remain!
Challenge - Create your own filter
Following the example above, try to create your own filters with different colors. Launch Replit
Finally, please think about and try to create a grey filter. We will talk about how to create a grey filter in the next section.