Activity 4 - Add effects to your song
Effects in EarSketch
Effects allows the producer to alter the sound of the audio clip into its own unique sound. Similar to how we used fitMedia()
to add new audio clips, we must use the setEffect()
function to define specific effects for each audio clip. It is important to note that multiple effects can be placed on the same track. This allows for the producer to combine multiple effects together. Earsketch has many effects built-in. To reference all the effects, visit this link
and make sure the Curriculum pane shows up on the right side of the screen.
Before we start adding effects to our song, let’s breakdown the pieces of the two setEffect
functions:
track
: The track number to which the effect is applied to. Note: To apply an effect to the Master track, use 0 as the value for track. The master track is where all the audio is collectively played together. This is an easy way to apply an effect to all the sounds being processed.type
: The specific effect being usedparameter
: The setting for the effect being usedvalue
: The value applied to the effectParameter
Add setEffect function to your song
Since we are more familiar with using effects, let’s add an effect to our song.
- On the API browser on the left, scroll to the
setEffect
API. - Insert a
setEffect
function call into your code, using the paste icon. - We need to replace the parameters with our own values. Put an integer value for
track
. Let’s put 2. - For the rest of the effect parameters, click on Open beside the
setEffect
API and you will see a link to “Every Effect Explained in Detail”. Click on it to open this section on the right side of your window.
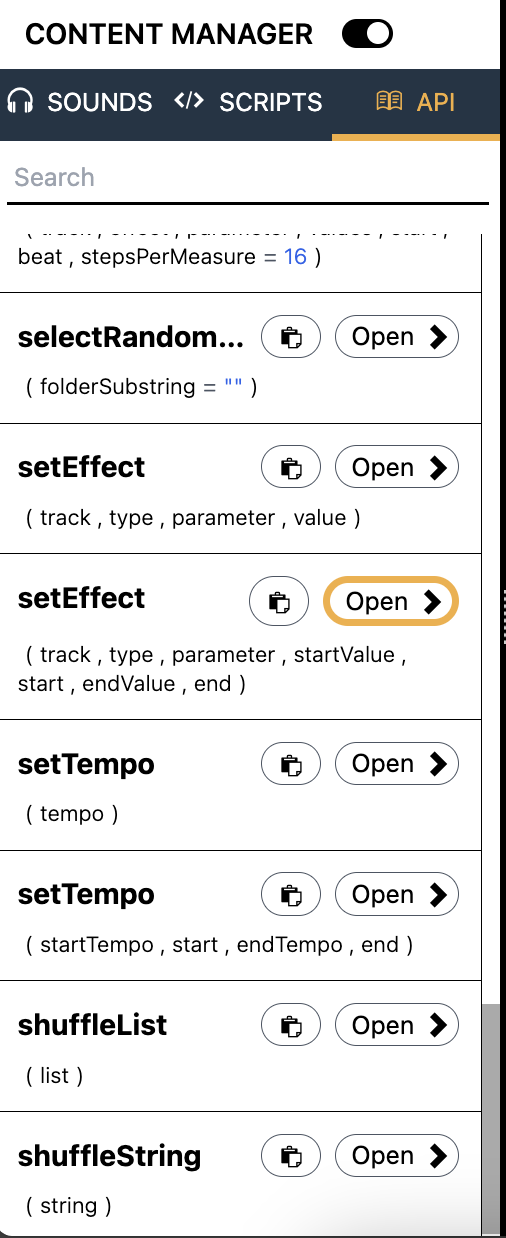
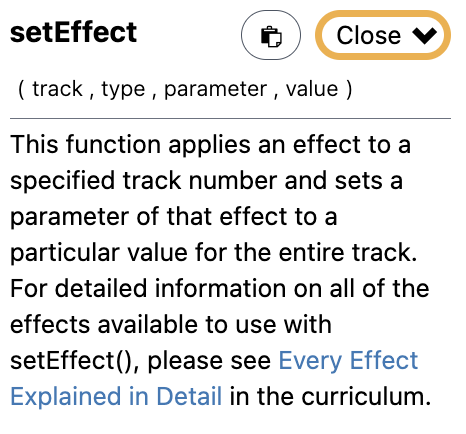
- You may notice that Earsketch has many different variations of effects. By default, you should see section 10.1 BANDPASS on the right. For now, we will use the DELAY from section 10.4 as
type
in oursetEffect
function call. - Now that we have chosen our
type
, we need anparameter
. Navigate to section 10.4 on the right to check out different parameters supported for DELAY. Here is a picture of that section. We recommend using the DELAY_FEEDBACK.
- For the final parameter, input a valid value. Since we have chosen DELAY_FEEDBACK, we must specify a number between -120.0 and -1.0 (as shown in the picture above). Example: Use -6.0 as your effect value for DELAY_FEEDBACK.
Your code should now look something like this:
from earsketch import *
setTempo(120)
fitMedia(HOUSE_DEEP_CRYSTALCHORD_001, 3, 1, 5)
fitMedia(HOUSE_DEEP_CRYSTALCHORD_002, 3, 5, 9)
# Section A
def sectionA(startMeasure, endMeasure):
fitMedia(RD_UK_HOUSE__AIRYPAD_1, 1, startMeasure, endMeasure)
fitMedia(HOUSE_MAIN_BEAT_002, 4, startMeasure, endMeasure)
fitMedia(HOUSE_ROADS_BASS_001, 5, startMeasure, endMeasure)
# Section B
def sectionB(startMeasure, endMeasure):
fitMedia(Y37_ORGAN_1, 2, startMeasure, endMeasure)
fitMedia(HOUSE_ROADS_BASS_001, 5, startMeasure, endMeasure)
fitMedia(RD_UK_HOUSE__ARPLEAD_1, 6, startMeasure, endMeasure)
sectionA(1, 9)
sectionB(9, 17)
sectionA(17, 25)
setEffect(2, DELAY, DELAY_FEEDBACK, -6.0)
setEffect(2, DELAY, DELAY_TIME, 1200.0)