Activity 9 - Adding Score, Levels, and Lives
Now, we will add some numbers to quantify our game! The easiest one to implement is the score. Create a text variable on the top left of the screen that displays Score:
and the value of a num variable that stores the player’s score.
this.scoreText = this.add.text(20, 10, "Score: " + this.score, {
font: "25px Arial",
fill: "white"
});
This line print score variable at the top left of the screen.
20
and10
is the x and y value"score" + this.score
is the text value
You can fill this line to this location after you create score value:
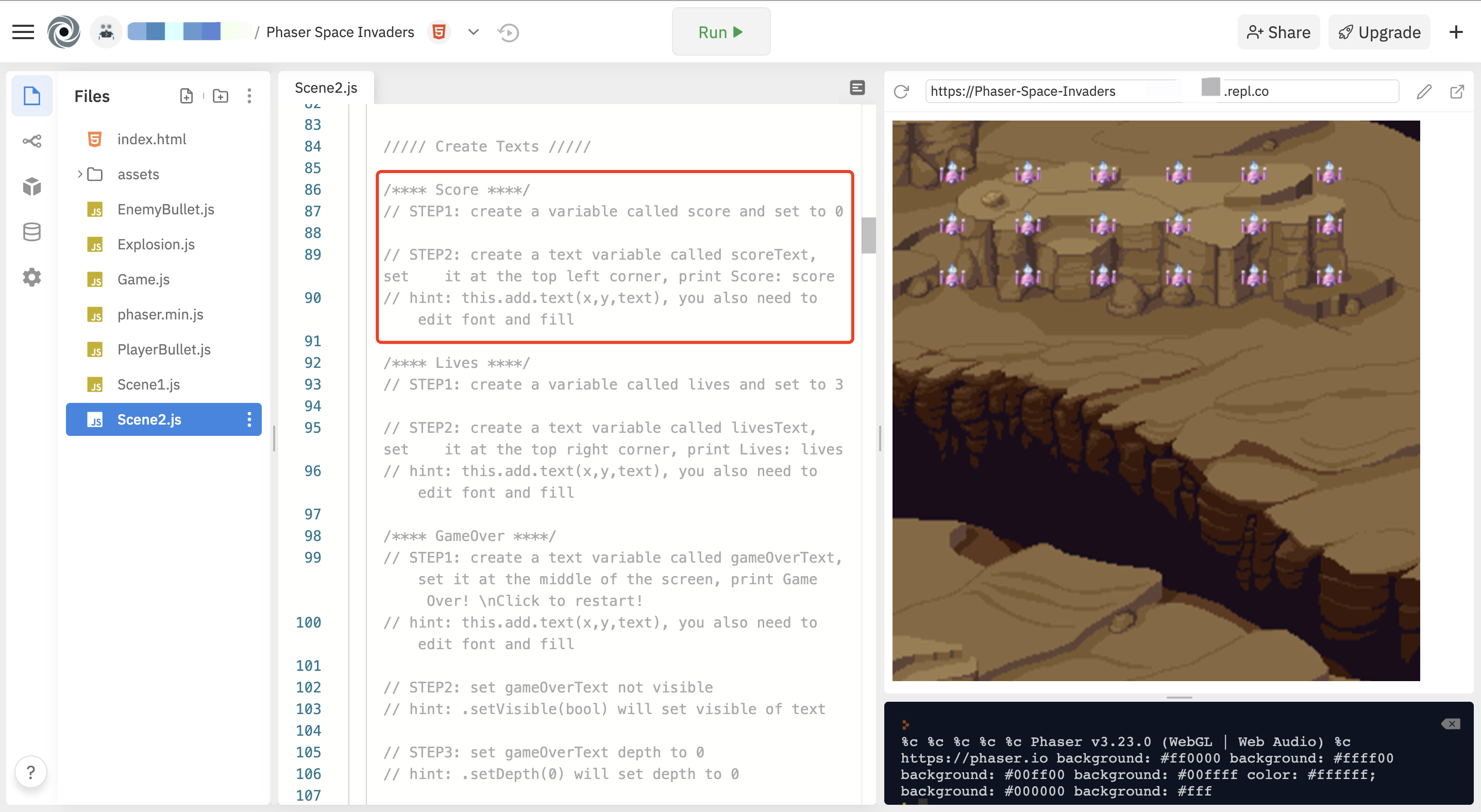
Just add a score variable and some text to the screen that displays “Score: ‘score variable’”. We will update the hitEnemy()
function so that the player will earn points to their score if they defeat an enemy:
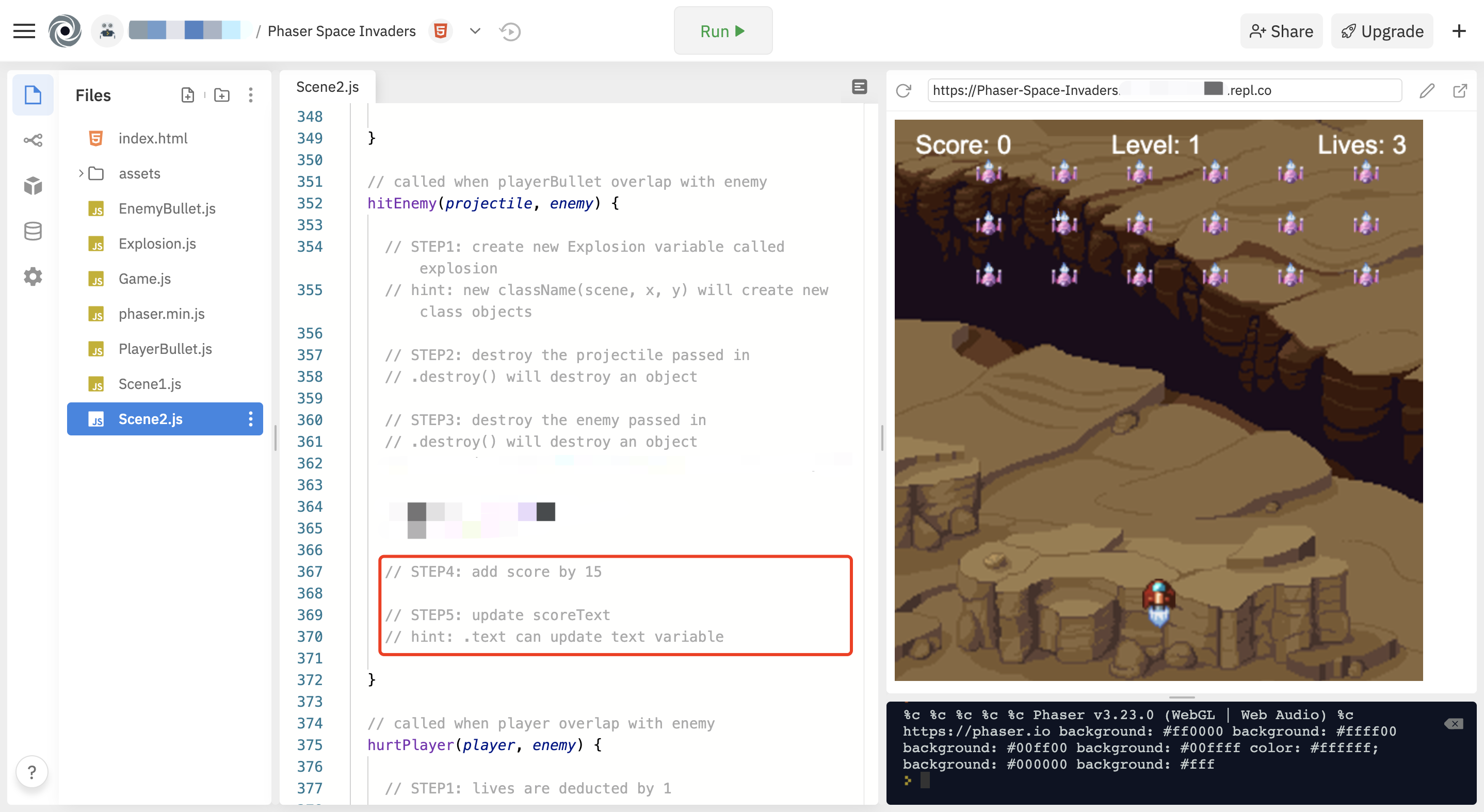
Now we will add levels. We will initialize it the same way we did for the score, but this time we will print it to the middle of the screen instead of the top left:
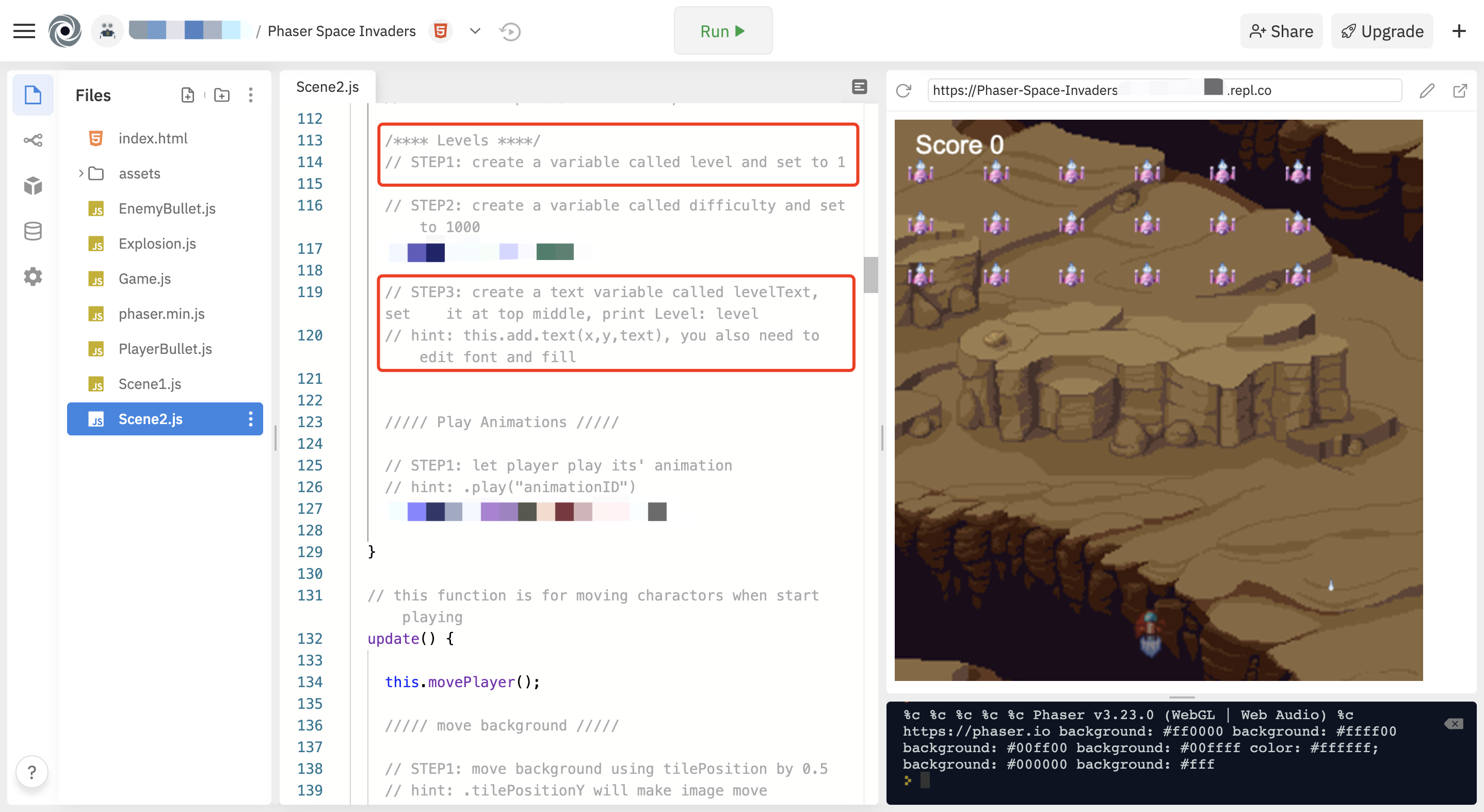
We will make levels pretty simple: whenever the player destroys all of the enemies, the next level will be reached, respawning all of the enemies. In order to make each level more difficult than the last, we will make the enemies shoot more often. To make the enemies shoot more often, let’s create a difficulty variable and use that when we make the enemies randomly shoot.
With this setup, by changing the difficulty variable, we change how often the enemies shoot. A lower difficulty will make the game harder because it will make the probability that an enemy shoots harder.
Now to make the level update when the player destroys all of the enemies, we will create a levelClear()
method that will update the difficulty variable, reset the enemy timer, create new enemies (by calling createEnemies()
), and update the level text on screen :
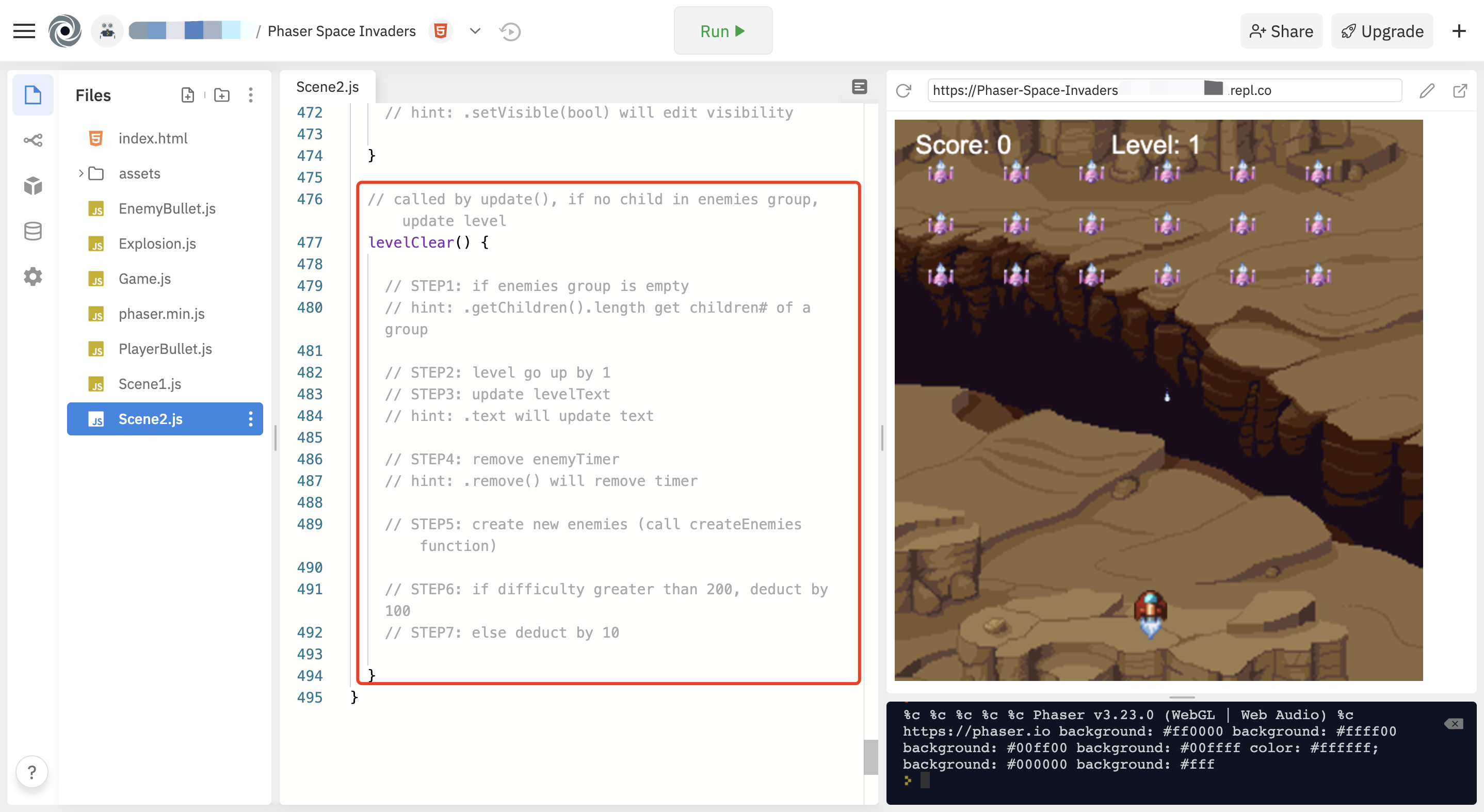
Note how we have to create a new timer every time we create new enemies or else the enemies and timer might not be in sync. Also, we recommend decrementing the difficulty variable less when we reach 200 because the difficulty ramps up much quicker at that point.
Don’t forgot to update levelClear() in update()
:
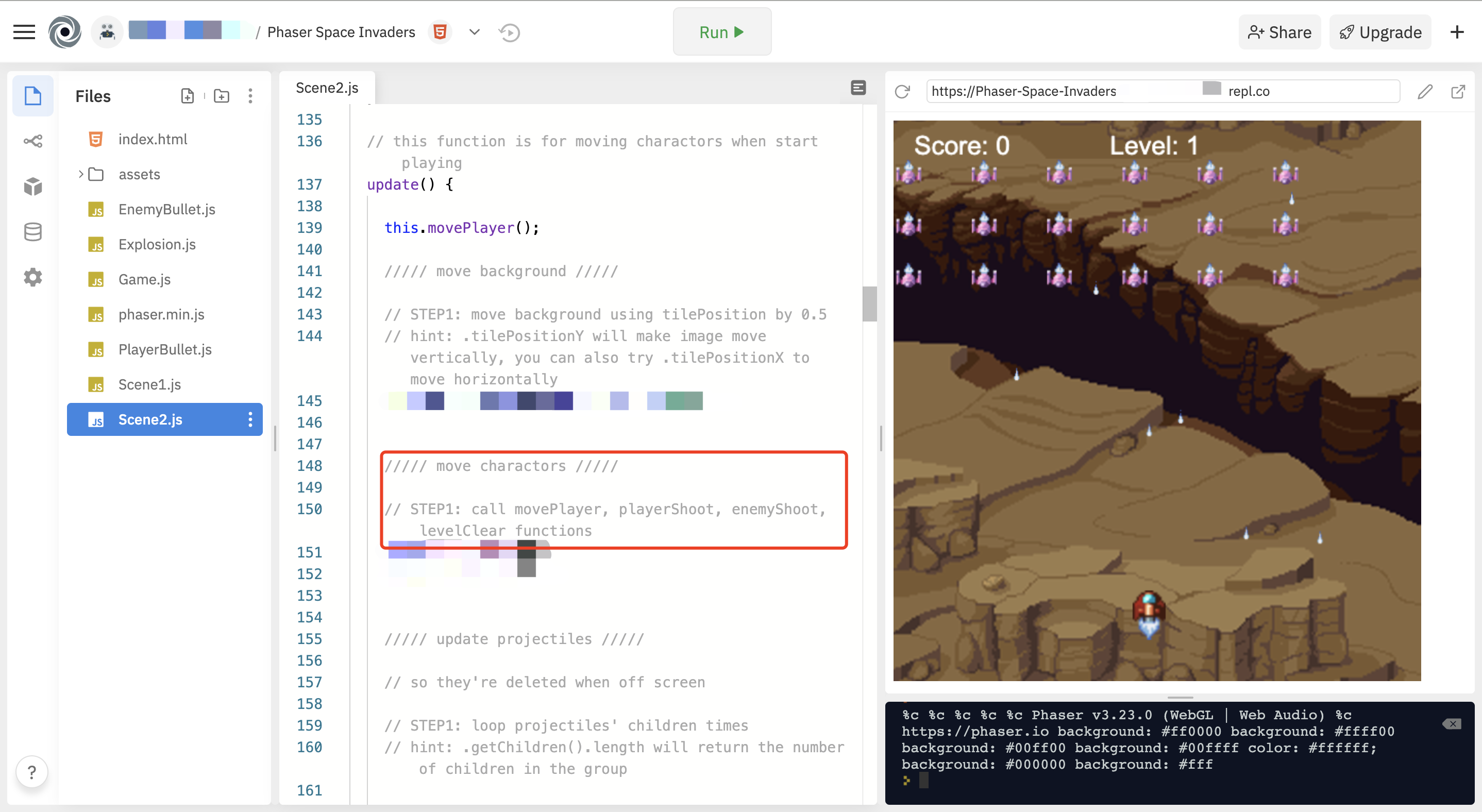
Now, we will add lives. We will again use a similar method to initialize it and put it on the top right of the screen:
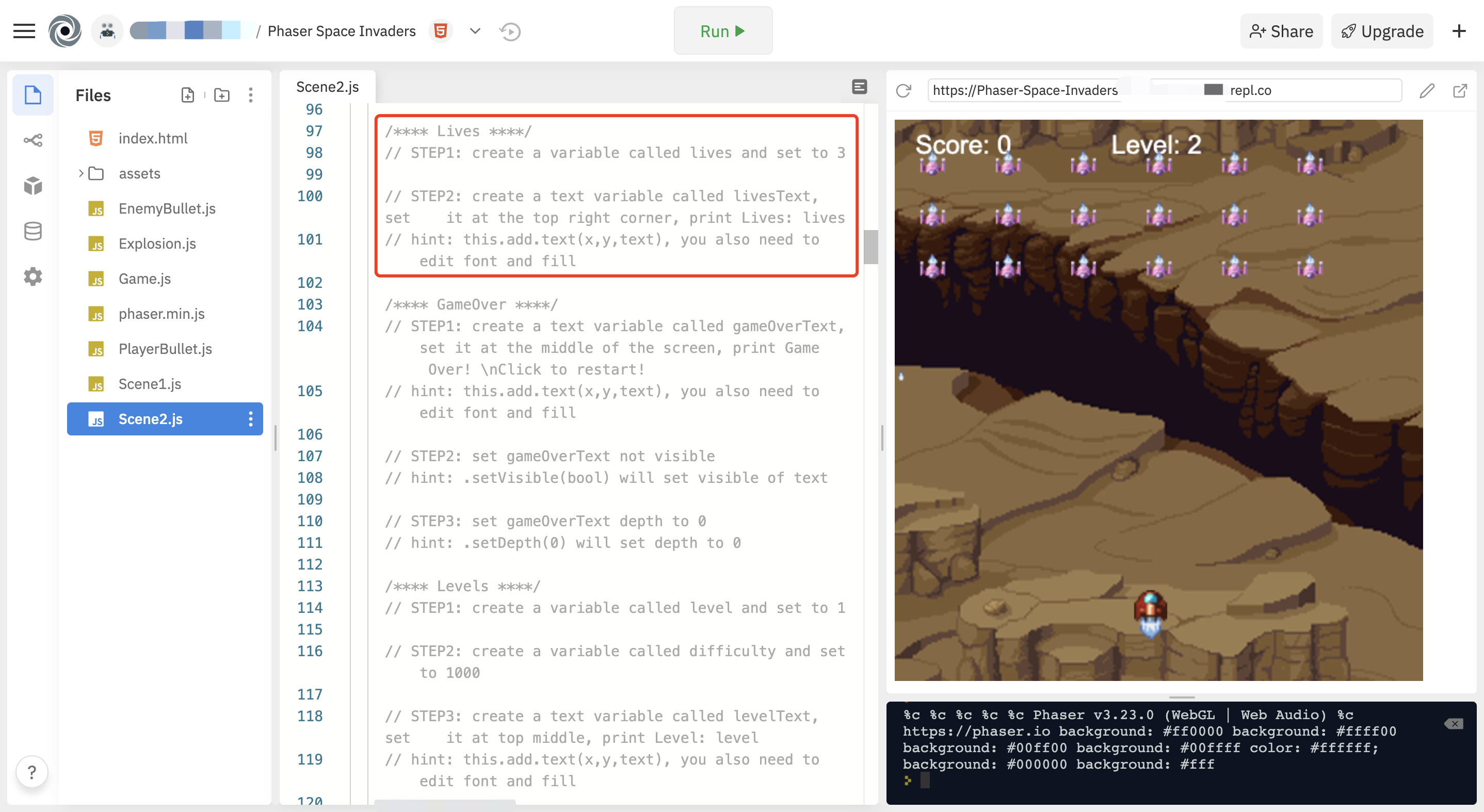
Now, in order to lose a life whenever you get hit, now let’s add this code to hurtPlayer()
:
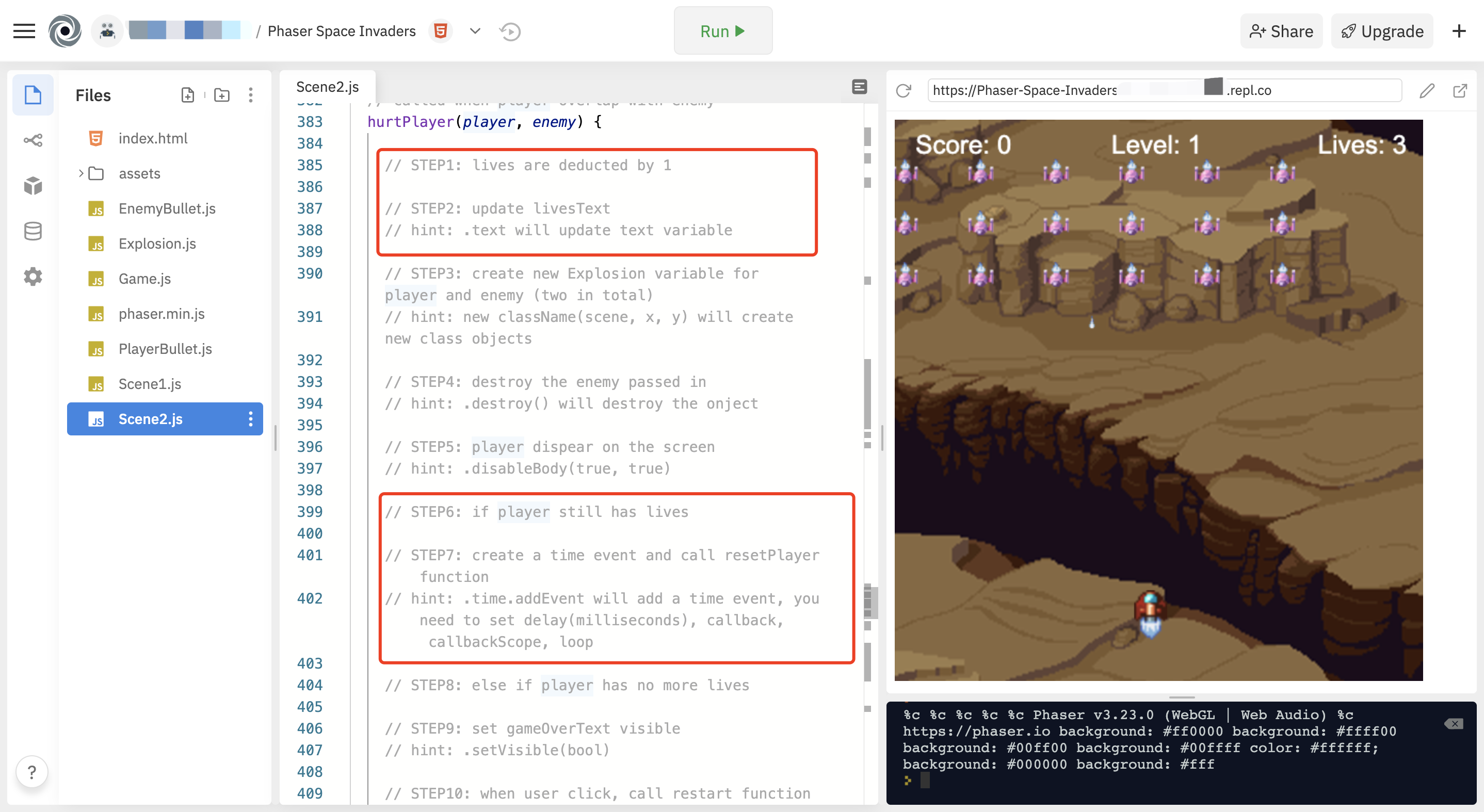
However, in order for the lives to mean anything, let’s make it a Game Over when the player. We will start by making some text that’s invisible on the screen in the create()
method:
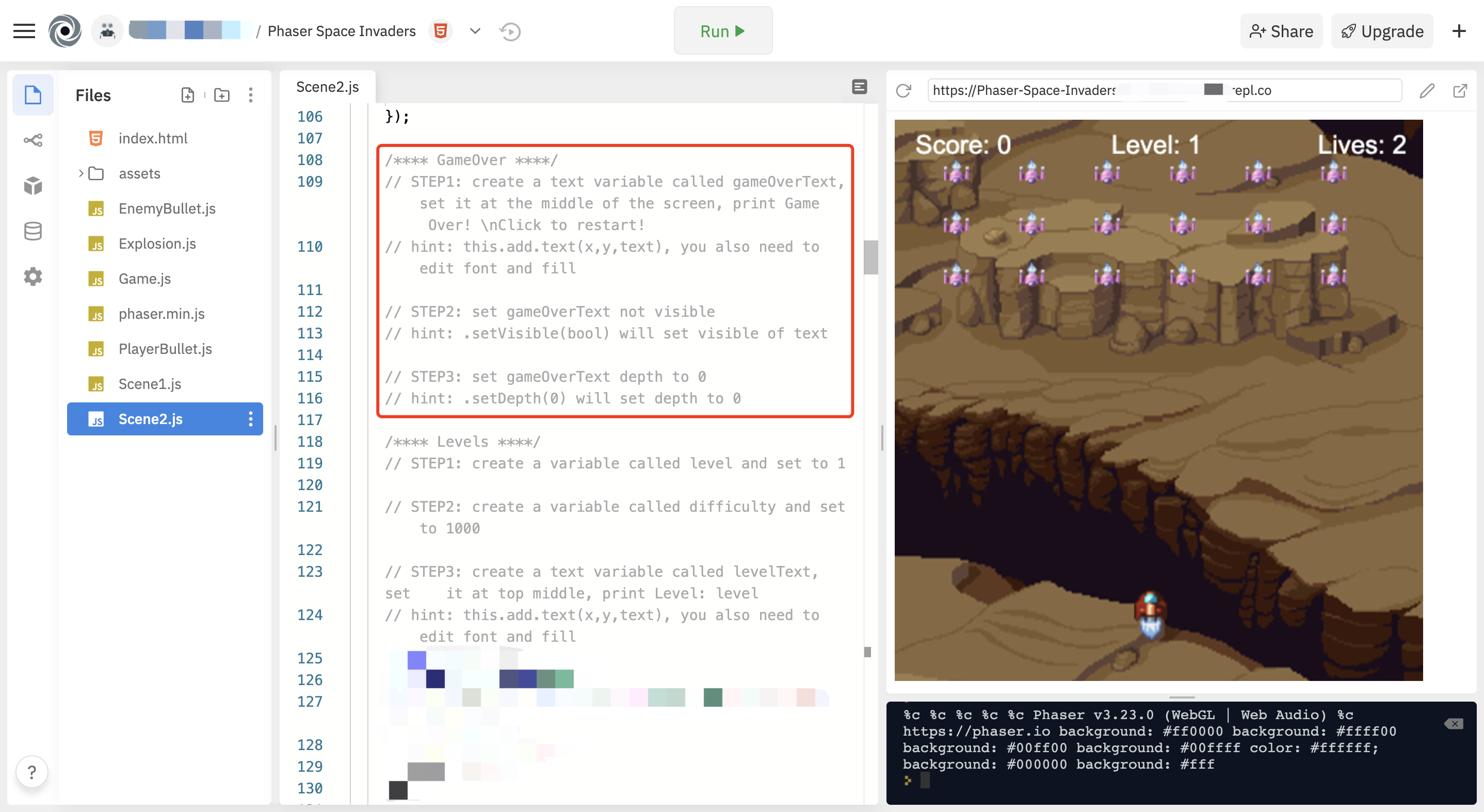
We will make this text visible when it is a game over. We will do this in the hurtPlayer()
method:
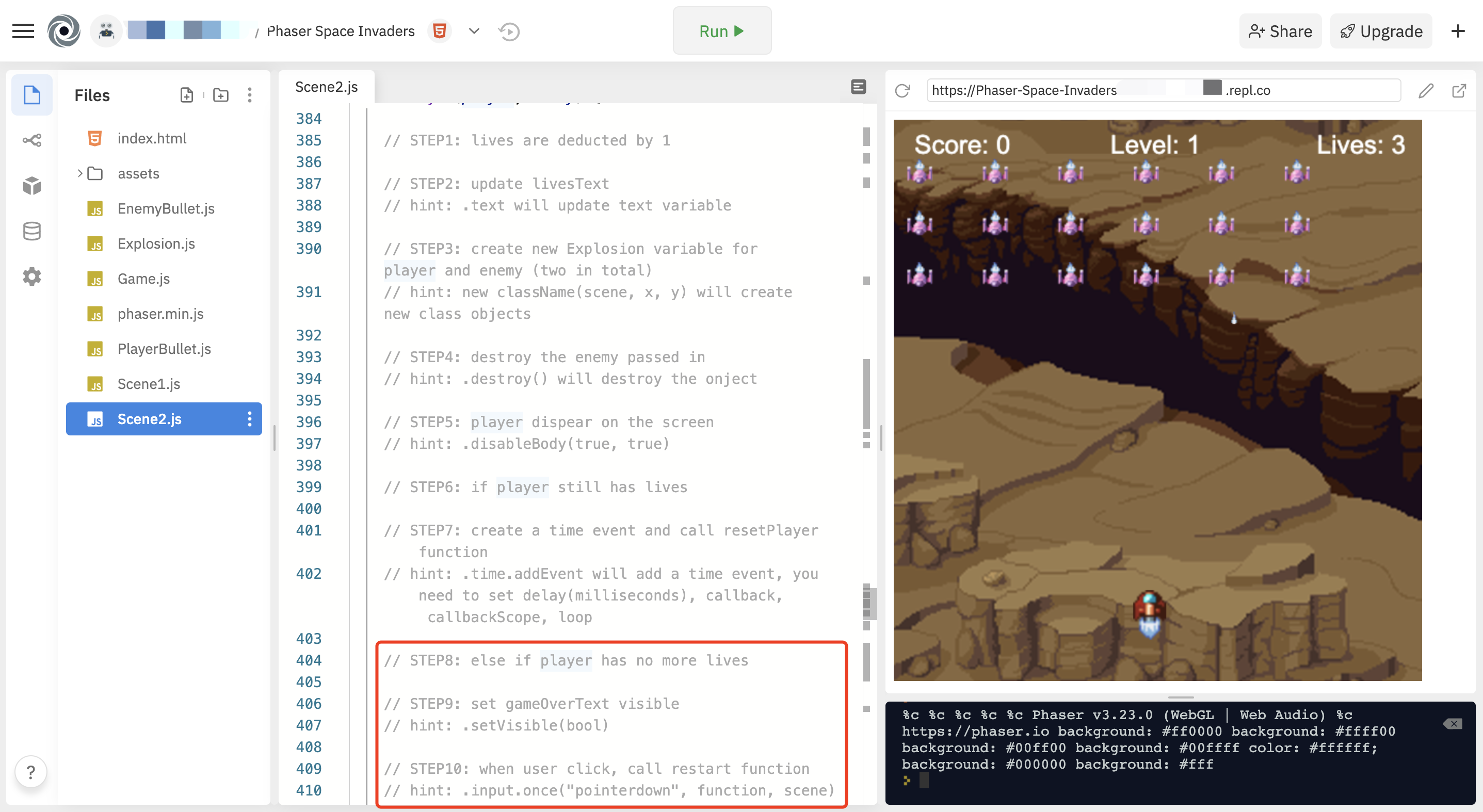
This makes the character not spawn, show the game over text, and restart the game if the player clicks on the screen.
Only thing now is that we need to create the restart()
method that this code refers to:
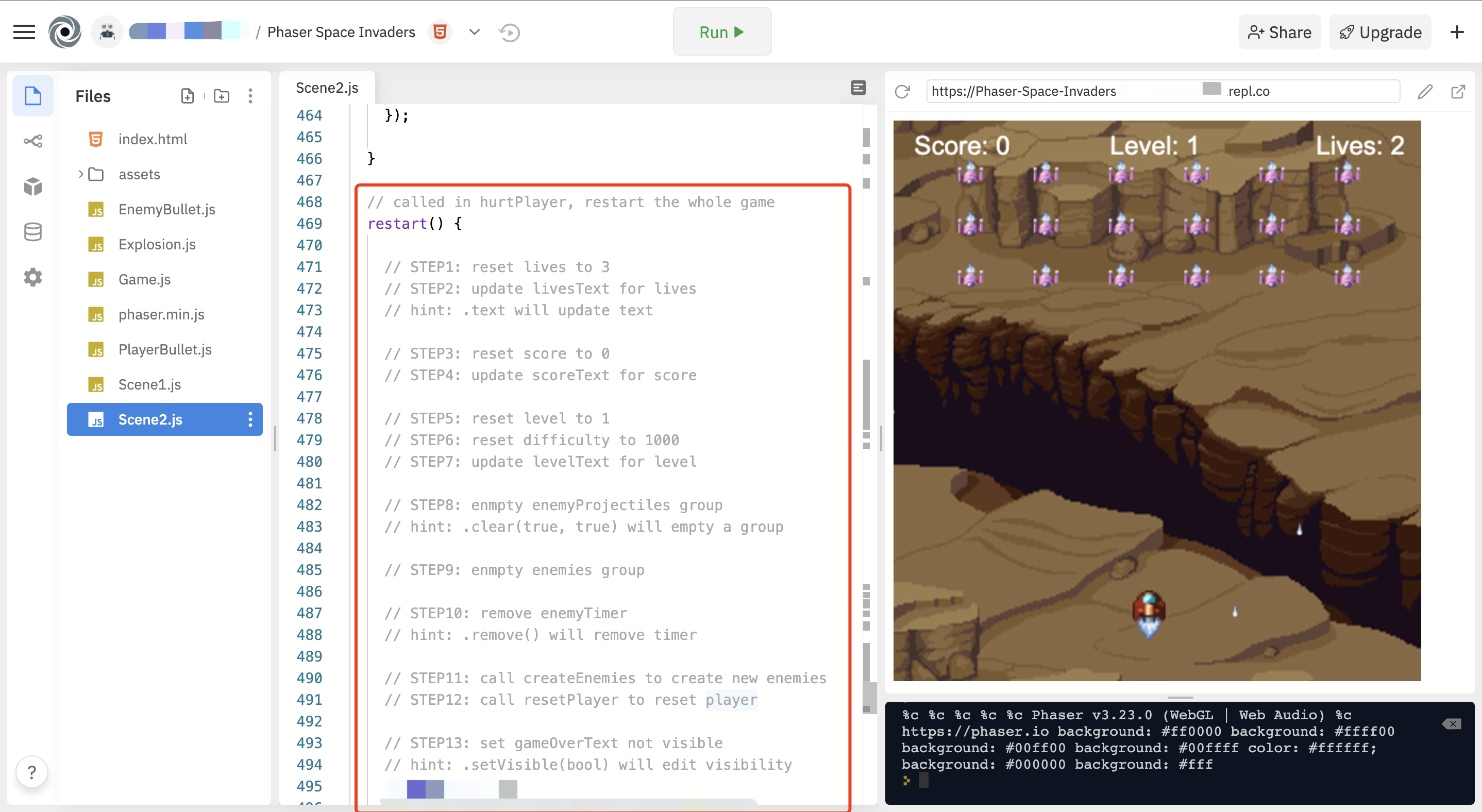
This resets everything to start the game over