Activity 3 - Add Keyboard Input to Move the Player
Now, let’s allow our ship to move when the player uses the keyboard. The first step is to create a cursor attribute in the create()
method in Scene2.js
:
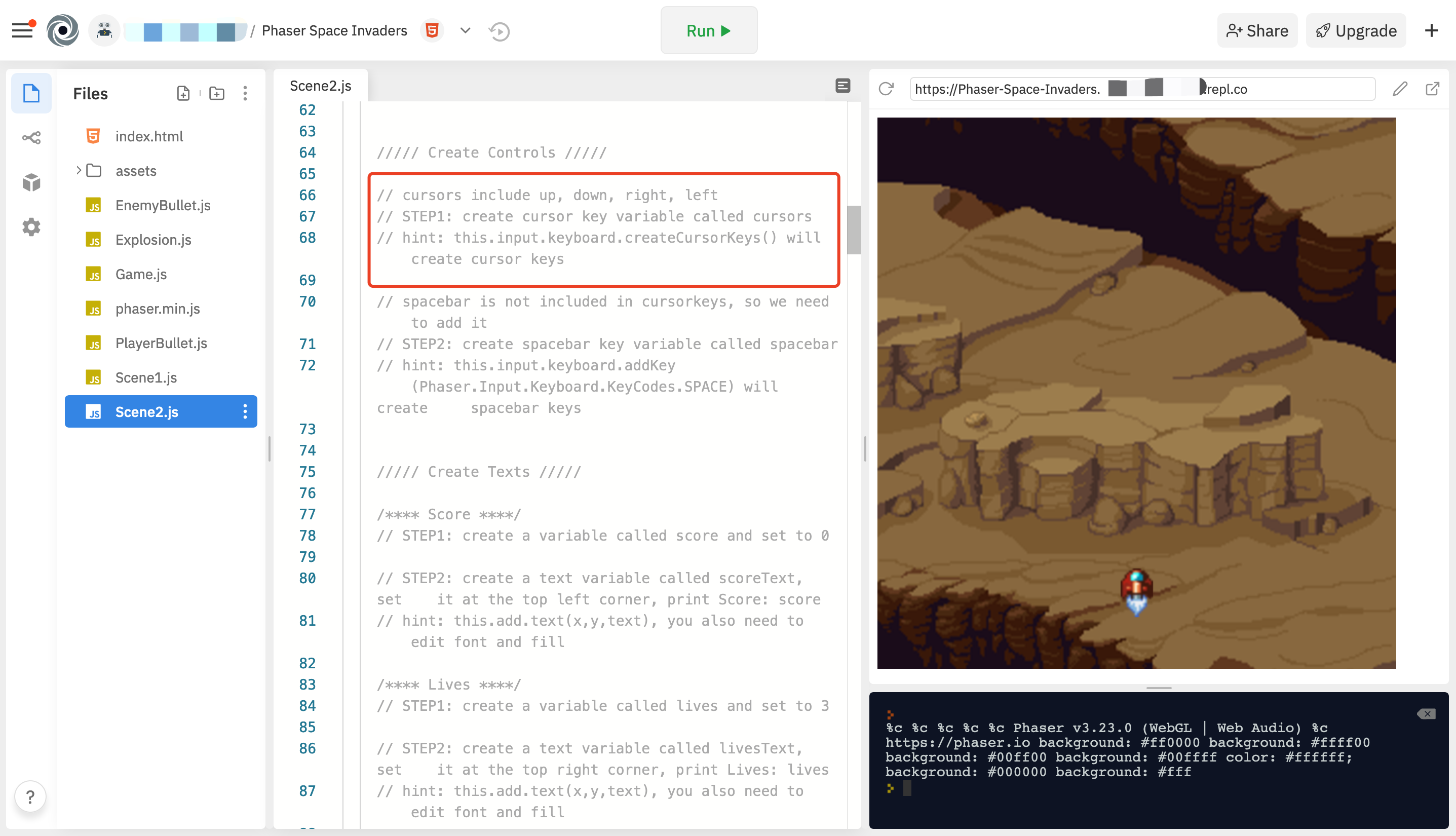
The most efficient way to create movement is to create a helper function that uses the cursor attribute to check whether the keyboard has been pressed. We have already made this function for you, and it is called movePlayer()
:
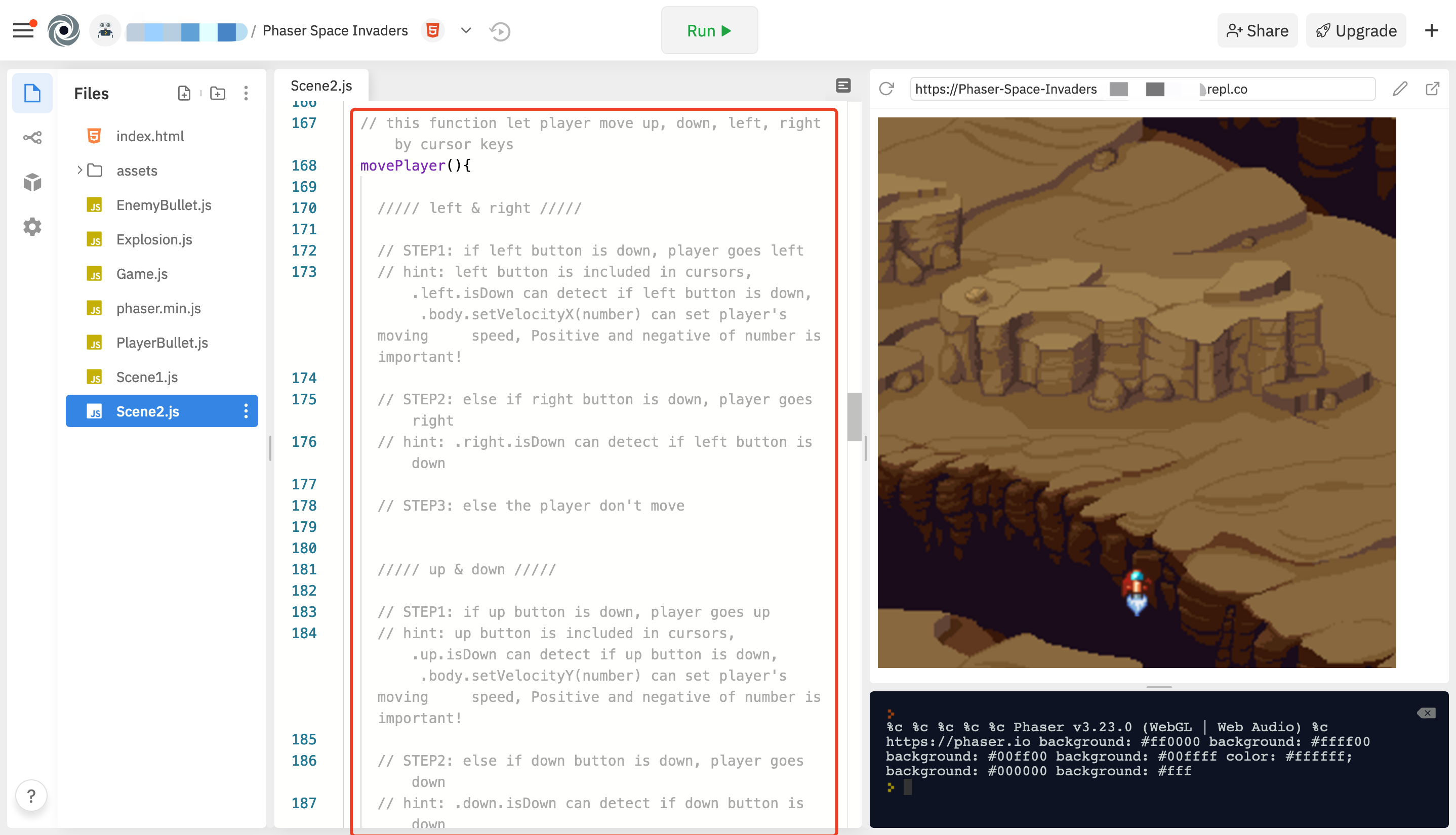
We then move the player with this function. We can call this function in the update()
method so that we check for movement during every update cycle (this has also already been done for you).
Now, go to the movePlayer()
method, and write code that will check for movement and move the player accordingly.
- We would recommend a movement speed of 200
- Use an if statement
- After you’ve made the
if
statements, you may find that your player is continuing to move even after you’ve stopped pressing on the keyboard. To fix this, think: when the player is not pressing the keyboard, what should the ship be doing?
Now test it out! You may notice one thing however; the player is able to move outside of the screen like this:
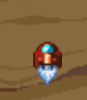
This happens because Phaser uses on an unlimited canvas that exists even outside of the screen’s window. We can fix this by restricting the player to the screen with one line of code:
this.player.body.setCollideWorldBounds(true); // player cannot go out of screen
Find the create()
method of Scene 2:
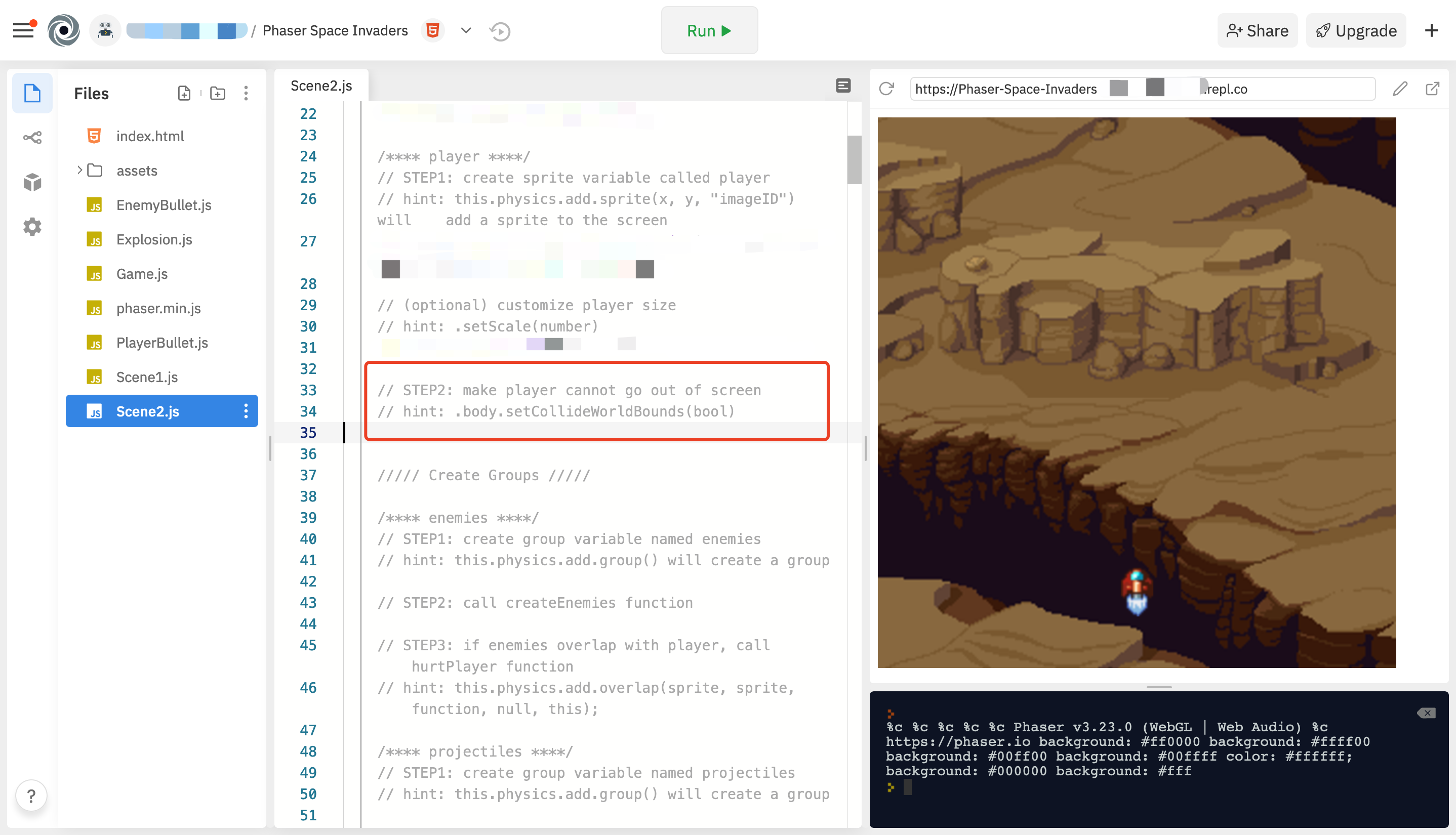
Now, that your player is bound within the screen, you should be able to move your ship like this:
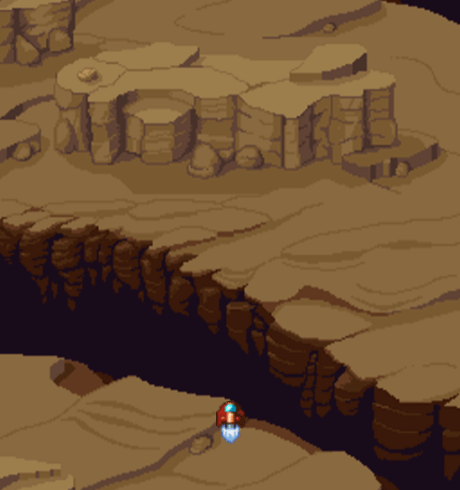