Classes and Objects
What is an Object and what is a Class?
An Object is a thing (noun) that has certain characteristics and can perform certain tasks. A Class is the blueprint or definition for that object when it is created.
An example is a Person. A Person is a kind of Class. A person can have certain characteristics that distinguish it from another person. It can have blue eyes, it can be 15 years old, etc. A person can also perform certain tasks. It can walk, it can talk, etc.
A C# Object is an instance of a C# Class. In this instance “Bea” is a “Person”. “Bea” has brown eyes. This is a property (or data field) of “Bea”. “Bea” can speak in English. “Bea” can speak in Spanish. These are methods that “Bea” can perform.
Creating a Class!
C# is an object-oriented programming language, which means everything in C# is associated with an object and a class (the blueprint for the object).
For starters, the line of code that we used to print statements from activity 1 is actually a method call from a predefined class called System
!
Console.WriteLine("Hello World");
Console
is a class that deals with user input and outputs.WriteLine()
is a method defined in the classConsole
.
Another built-in class we have interacted with in the previous exercises is string
. The string
class defines a set of rules on how a list of characters should behave.
With the following line of code, we created a string
object called name
using the rules defined in the string
class:
string name = "Patrick";
Console
, and string
are predefined classes in C#. However, we are not limited to these predefined classes, and we can actually create our own data type by writing a class! This is useful for coders to create specific objects to have certain attributes and behaviors. Having access to these user-defined types allows us to build distinct programs.
Let’s learn about the different parts in a class below:
Class
A class is a blueprint or prototype of a new type of object. In general, a class contains three important parts:

Element | Description | Example |
---|---|---|
data fields/instance variables | variables an object of this class has access to that describe the object | For example, a Person class could have fields eyeColor, age, height. |
constructor | method called automatically when an object from this class is created, constructors have the same name as the class | There can be more than one constructor per class |
methods | methods for the object of this class to perform certain tasks | Person class could have methods talk and walk. |
For example:
public class Person{
// (1) data fields/instance variables
private String name; // example
private int age;
private int height;
// (2) constructor - constructors have the same name as the class
public Person()
{
name = "Bea";
age = 29;
height = 167;
}
// (2) constructor - you can have more than one
public Person( String nameInput, int ageInput, int heightInput)
{
name = nameInput;
age = ageInput;
height = heightInput;
}
// (3) methods
public void talk()
{
Console.WriteLine($"Hello from {name}");
}
}
Try it Out 🐥!
Let’s make a Bird
class to represent Patrick 🐥 and all of his Bird friends by following the steps below!
First, we start by defining the class name in the format public
class
name
.
public class Bird{}
Second, let’s declare all the fields of the Bird class: species, name, hobby, age, loveMusic.
Each field is declared in the format: access specifier
data type
name
;
.
We declare all 5 fields as
private
in the classBird
. This ensures these fields can only be accessed within this class.Let’s identify the appropriate type for each field:
species
should be aString
that stores for exmaple: “duck”, “swan”, “owl”.name
should be aString
that stores for example: “Patrick”.hobby
should be aString
that stores for example: “play basketball”.age
should be anint
that stores for example: 25.loveMusic
should be abool
that stores either true or false.
For example, to declare species
as a private field of class Bird
, you would put private String species;
.
- Finish creating the other 4 fields of the Bird class!
Third, let’s create the constructor for the class Bird
.
Usually, constructor is the method that initializes value to all the fields in a class. It has the format public
class name
(parameter)
. Since we have 5 fields in this class, the constructor will take in 5 parameter/inputs.
public Bird(string speciesInput, string nameInput, string hobbyInput, int ageInput, bool loveMusicInput){
// constructor body
}
In the constructor body, we need to initialize all the instance variables, by assign each variables to its initial values:
species = speciesInput;
name = nameInput;
hobby = hobbyInput;
age = ageInput;
loveMusic = loveMusicinput;
Lastly, let’s create some methods for the class Bird.
We are gonna create 6 methods for this class!
getSpecies();
// return species of the birdgetName();
// return name of the birdgetHobby();
// return hobby of the birdgetAge();
// return age of the birdgetLoveMusic();
// return whether the bird loves musicToString();
// return information in a string
Try to write out the first 5 methods with what you learned from the previous exercise about writing methods!
After, let’s write the ToString()
method together!
ToString()
is a method that returns the string
representation of the object.
We will return a String that include all fields of the Bird
: name, age, species, hobby, loveMusic by calling the 5 methods you just implemented!
public string ToString(){
// initialize variable info to have empty String
string info = "";
// append information to the String in the format:
// Hi, my name is [name]. I am a [age] year old [species] who likes to [hobby].
info = info + "Hi, my name is " + getName() +
". I am a " + getAge() + " year old " + getSpecies() +
" who likes to " + getHobby() + ". ";
// if the Bird likes music, append the String "I also like music very much!" to info
if(getLoveMusic()){
info = info + "I also like music very much!";
}else{
info = info + "However, I do not like music!";
}
// return the full string
return info;
}
If you correctly implemented the Bird
class, you will see the following message when you click Run
:
Congratulations! You correctly implemented the Bird Class :)
If there is still something incomplete or incorrect when you click Run
:
- You might get an error such as
todo: fill this in
. - Or, you will see the message,
Something is still not quite right!
.
Creating Birds 🐥!
Congratulations! You just wrote you first C# class Bird
class. Now let’s learn to write a program that uses Bird
objects!
We defined the Bird
class to have the following attributes (instance variables) and behaviors (methods):
To create a new object of a particular class, we call the constructor of that class in the format class name
variable name
=
new
constructor call
.
Recall that the constructor of Bird
class is the following:
public Bird(string speciesInput, string nameInput, string hobbyInput, int ageInput, bool loveMusicInput);
Hence, we can create a Bird with these attributes (species - duck; name - Patrick; hobby - hangout with friends; age - 15; loveMusic - true) with this line of code.
Bird patrick = new Bird("duck", "Patrick", "hangout with friends", 15, true);
Try it out yourself and create multiple Birds of different species 🐦🐤🐔🐧!
Next, let's call the `ToString()` method on these `Bird` objects we created to print the information of our friends. You can do either of the following:
- Print the return value of
ToString()
method. (i.e.Console.WriteLine(patrick.ToString());
) - Directly print the
Bird
object, which promptsToString()
to be called in the background. (i.e.Console.WriteLine(patrick);
).
Try it out and print out all the information of the bird friends you created 🐦🐤🐔🐧!
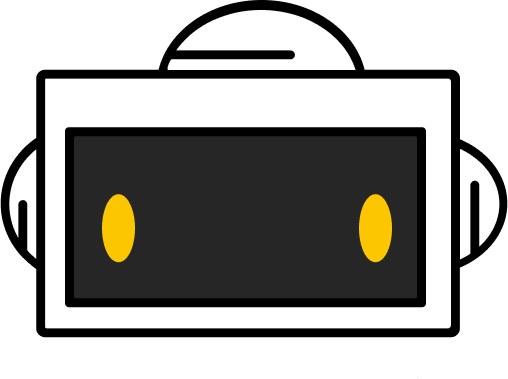
You did it!
Workshop complete